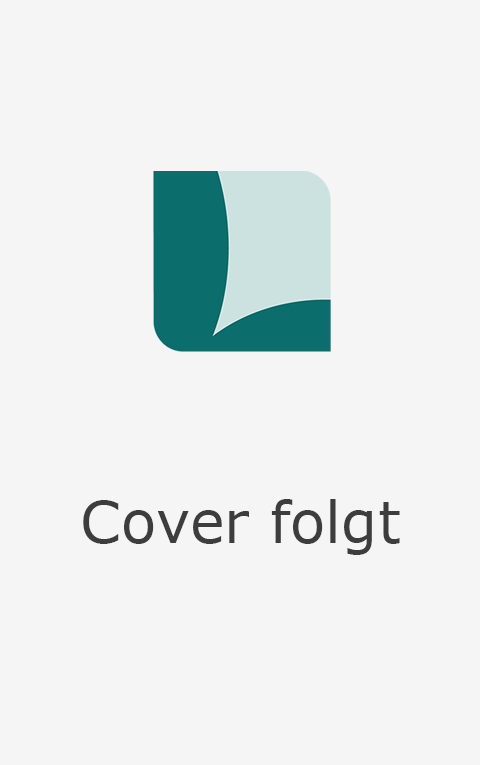
Introduction to Assembly Language Programming (eBook)
713 Seiten
Springer New York (Verlag)
978-0-387-27155-2 (ISBN)
The book requires only some basic experience with a structured, high-level language.
Topics and Features:
Introduces assembly language so that readers can benefit from learning its utility with both CISC and RIS
- Employs the freely available NASM assembler, which works with both Microsoft Windows and Linux operating systems [NEW].
- Contains a revised chapter on "Basic Computer Organization" [ NEW].
- Uses numerous examples, hands-on exercises, programming code analyses and challenges, and chapter summaries.
- Incorporates full new chapters on recursion, protected-mode interrupt processing, and floating-point instructions [NEW].
Assembly language programming is part of several undergraduate curricula in computer science, computer engineering, and electrical engineering. In addition, this newly revised text/reference can be used as an ideal companion resource in a computer organization course or as a resource for professional courses.
Assembly language continues to hold a core position in the programming world because of its similar structure to machine language and its very close links to underlying computer-processor architecture and design. These features allow for high processing speed, low memory demands, and the capacity to act directly on the system's hardware. This completely revised second edition of the highly successful Introduction to Assembly Language Programming introduces the reader to assembly language programming and its role in computer programming and design. The focus is on providing readers with a firm grasp of the main features of assembly programming, and how it can be used to improve a computer's performance. The revised edition covers a broad scope of subjects and adds valuable material on protected-mode Pentium programming, MIPS assembly language programming, and use of the NASM and SPIM assemblers for a Linux orientation. All of the language's main features are covered in depth. The book requires only some basic experience with a structured, high-level language.Topics and Features: Introduces assembly language so that readers can benefit from learning its utility with both CISC and RISC processors [ NEW ].- Employs the freely available NASM assembler, which works with both Microsoft Windows and Linux operating systems [ NEW ].- Contains a revised chapter on "e;Basic Computer Organization"e; [ NEW].- Uses numerous examples, hands-on exercises, programming code analyses and challenges, and chapter summaries.- Incorporates full new chapters on recursion, protected-mode interrupt processing, and floating-point instructions [ NEW ].Assembly language programming is part of several undergraduate curricula in computer science, computer engineering, and electrical engineering. In addition, this newly revised text/reference can be used as an ideal companion resource in a computer organization course or as a resource for professional courses.
Preface 7
Contents 13
PART I Overview 24
1 Introduction 26
1.1 A User’s View of Computer Systems 27
1.2 What Is Assembly Language? 28
1.3 Advantages of High-Level Languages 31
1.4 Why Program in the Assembly Language? 32
1.5 Typical Applications 33
1.6 Why Learn the Assembly Language? 34
1.7 Performance: C Versus Assembly Language 35
1.7.1 Multiplication Algorithm 35
1.8 Summary 37
1.9 Exercises 37
1.10 Programming Exercises 38
1.11 Program Listings 38
2 Basic Computer Organization 42
2.1 Basic Components of a Computer System 43
2.2 The Processor 45
2.2.1 The Execution Cycle 45
2.2.2 The System Clock 46
2.3 Number of Addresses 47
2.3.1 Three-Address Machines 47
2.3.2 Two-Address Machines 48
2.3.3 One-Address Machines 49
2.3.4 Zero-Address Machines 49
2.3.5 The Load/Store Architecture 49
2.3.6 Processor Registers 50
2.4 Flow of Control 51
2.4.1 Branching 51
2.4.2 Procedure Calls 53
2.5 Memory 55
2.5.1 Two Basic Memory Operations 56
2.5.2 Types of Memory 58
2.5.3 Storing Multibyte Data 60
2.6 Input/Output 61
2.7 Performance: Effect of Data Alignment 64
2.8 Summary 66
2.9 Exercises 66
PART II Pentium Assembly Language 68
3 The Pentium Processor 70
3.1 The Pentium Processor Family 70
3.2 The Pentium Registers 72
3.2.1 Data Registers 73
3.2.2 Pointer and Index Registers 73
3.2.3 Control Registers 74
3.2.4 Segment Registers 76
3.3 Protected-Mode Memory Architecture 76
3.3.1 Segment Registers 77
3.3.2 Segment Descriptors 78
3.3.3 Segment Descriptor Tables 80
3.3.4 Segmentation Models 81
3.4 Real-Mode Memory Architecture 82
3.5 Mixed-Mode Operation 85
3.6 Which Segment Register to Use 86
3.7 Initial State 87
3.8 Summary 88
3.9 Exercises 88
4 Overview of Assembly Language 90
4.1 Assembly Language Statements 91
4.2 Data Allocation 92
4.3 Where Are the Operands? 97
4.3.1 Register Addressing Mode 98
4.3.2 Immediate Addressing Mode 98
4.3.3 Direct Addressing Mode 99
4.3.4 Indirect Addressing Mode 100
4.4 Data Transfer Instructions 101
4.4.1 The MOV Instruction 101
4.4.2 Ambiguous Moves 102
4.4.3 The XCHG Instruction 102
4.4.4 The XLAT Instruction 103
4.5 Overview of Assembly Language Instructions 103
4.5.1 Simple Arithmetic Instructions 103
4.5.2 Conditional Execution 106
4.5.3 Iteration Instruction 109
4.5.4 Logical Instructions 110
4.5.5 Shift Instructions 112
4.5.6 Rotate Instructions 114
4.6 Defining Constants 116
4.6.1 The EQU Directive 116
4.6.2 The %assign Directive 117
4.6.3 The %define Directive 117
4.7 Macros 118
4.8 Illustrative Examples 121
4.9 Performance: When to Use XLAT Instruction 131
4.9.1 Experiment 1 131
4.9.2 Experiment 2 133
4.10 Summary 133
4.11 Exercises 134
4.12 Programming Exercises 136
5 Procedures and the Stack 140
5.1 What Is a Stack? 141
5.2 Pentium Implementation of the Stack 141
5.3 Stack Operations 143
5.3.1 Basic Instructions 143
5.3.2 Additional Instructions 144
5.4 Uses of the Stack 146
5.4.1 Temporary Storage of Data 146
5.4.2 Transfer of Control 147
5.4.3 Parameter Passing 147
5.5 Procedures 147
5.6 Pentium Instructions for Procedures 149
5.6.1 How Is Program Control Transferred? 150
5.6.2 The ret Instruction 151
5.7 Parameter Passing 151
5.7.1 Register Method 152
5.7.2 Stack Method 155
5.7.3 Preserving Calling Procedure State 158
5.7.4 Which Registers Should Be Saved 159
5.7.5 ENTER and LEAVE Instructions 160
5.7.6 Illustrative Examples 161
5.8 Handling a Variable Number of Parameters 169
5.9 Local Variables 173
5.10 Multiple Source Program Modules 179
5.11 Performance: Procedure Overheads 182
5.11.1 Procedure Overheads 182
5.11.2 Local Variable Overhead 183
5.12 Summary 183
5.13 Exercises 185
5.14 Programming Exercises 186
6 Addressing Modes 190
6.1 Introduction 190
6.2 Memory Addressing Modes 192
6.2.1 Based Addressing 194
6.2.2 Indexed Addressing 194
6.2.3 Based-Indexed Addressing 196
6.3 Illustrative Examples 196
6.4 Arrays 203
6.4.1 One-dimensional Arrays 203
6.4.2 Multidimensional Arrays 204
6.4.3 Examples of Arrays 207
6.5 Performance: Usefulness of Addressing Modes 209
6.6 Summary 213
6.7 Exercises 214
6.8 Programming Exercises 215
7 Arithmetic Flags and Instructions 220
7.1 Status Flags 220
7.1.1 The Zero Flag 221
7.1.2 The Carry Flag 223
7.1.3 The Over.ow Flag 226
7.1.4 The Sign Flag 228
7.1.5 The Auxiliary Flag 229
7.1.6 The Parity Flag 231
7.1.7 Flag Examples 232
7.2 Arithmetic Instructions 234
7.2.1 Multiplication Instructions 234
7.2.2 Division Instructions 238
7.3 Illustrative Examples 241
7.3.1 PutInt8 Procedure 241
7.3.2 GetInt8 Procedure 244
7.4 Multiword Arithmetic 247
7.4.1 Addition and Subtraction 247
7.4.2 Multiplication 248
7.4.3 Division 252
7.5 Performance: Multiword Multiplication 255
7.6 Summary 256
7.7 Exercises 257
7.8 Programming Exercises 258
8 Selection and Iteration 262
8.1 Unconditional Jump 262
8.2 Compare Instruction 265
8.3 Conditional Jumps 267
8.3.1 Jumps Based on Single Flags 267
8.3.2 Jumps Based on Unsigned Comparisons 269
8.3.3 Jumps Based on Signed Comparisons 270
8.3.4 A Note on Conditional Jumps 272
8.4 Looping Instructions 273
8.5 Implementing High-Level Language Decision Structures 275
8.5.1 Selective Structures 275
8.5.2 Iterative Structures 278
8.6 Illustrative Examples 280
8.7 Indirect Jumps 286
8.7.1 Multiway Conditional Statements 289
8.8 Summary 289
8.9 Exercises 291
8.10 Programming Exercises 292
9 Logical and Bit Operations 294
9.1 Logical Instructions 295
9.1.1 The and Instruction 295
9.1.2 The or Instruction 298
9.1.3 The xor Instruction 299
9.1.4 The not Instruction 301
9.1.5 The test Instruction 301
9.2 Shift Instructions 301
9.2.1 Logical Shift Instructions 302
9.2.2 Arithmetic Shift Instructions 304
9.2.3 Why Use Shifts for Multiplication and Division? 305
9.2.4 Doubleshift Instructions 306
9.3 Rotate Instructions 307
9.3.1 RotateWithout Carry 307
9.3.2 Rotate Through Carry 308
9.4 Logical Expressions in High-Level Languages 309
9.4.1 Representation of Boolean Data 309
9.4.2 Logical Expressions 309
9.4.3 Bit Manipulation 310
9.4.4 Evaluation of Logical Expressions 311
9.5 Bit Instructions 313
9.5.1 Bit Test and Modify Instructions 314
9.5.2 Bit Scan Instructions 314
9.6 Illustrative Examples 314
9.7 Summary 320
9.8 Exercises 320
9.9 Programming Exercises 322
10 String Processing 324
10.1 String Representation 324
10.1.1 Explicitly Storing String Length 325
10.1.2 Using a Sentinel Character 326
10.2 String Instructions 326
10.2.1 Repetition Pre.xes 327
10.2.2 Direction Flag 328
10.2.3 String Move Instructions 329
10.2.4 String Compare Instruction 332
10.2.5 Scanning a String 334
10.3 Illustrative Examples 335
10.4 Testing String Procedures 344
10.5 Performance: Advantage of String Instructions 346
10.6 Summary 347
10.7 Exercises 348
10.8 Programming Exercises 349
11 ASCII and BCD Arithmetic 352
11.1 ASCII and BCD Representations of Numbers 353
11.1.1 ASCII Representation 353
11.1.2 BCD Representation 353
11.2 Processing in ASCII Representation 354
11.2.1 ASCII Addition 355
11.2.2 ASCII Subtraction 356
11.2.3 ASCII Multiplication 357
11.2.4 ASCII Division 357
11.2.5 Example: Multidigit ASCII Addition 358
11.3 Processing Packed BCD Numbers 359
11.3.1 Packed BCD Addition 359
11.3.2 Packed BCD Subtraction 361
11.3.3 Example: Multibyte Packed BCD Addition 361
11.4 Performance: Decimal Versus Binary Arithmetic 363
11.5 Summary 364
11.6 Exercises 365
11.7 Programming Exercises 367
PART III MIPS Assembly Language 368
12 MIPS Processor 370
12.1 Introduction 370
12.2 Evolution of CISC Processors 372
12.3 RISC Design Principles 374
12.4 MIPS Architecture 377
12.5 Summary 381
12.6 Exercises 382
13 MIPS Assembly Language 384
13.1 MIPS Instruction Set 384
13.1.1 Instruction Format 385
13.1.2 Data Transfer Instructions 386
13.1.3 Arithmetic Instructions 387
13.1.4 Logical Instructions 391
13.1.5 Shift Instructions 392
13.1.6 Rotate Instructions 393
13.1.7 Comparison Instructions 394
13.1.8 Branch and Jump Instructions 394
13.2 SPIM Simulator 396
13.2.1 SPIM System Calls 396
13.2.2 SPIM Assembler Directives 398
13.2.3 MIPS Program Template 400
13.3 Illustrative Examples 401
13.4 Procedures 409
13.5 Stack Implementation 414
13.6 Summary 416
13.7 Exercises 417
13.8 Programming Exercises 418
PART IV Interrupt Processing 422
14 Protected-Mode Interrupt Processing 424
14.1 Introduction 424
14.2 A Taxonomy of Interrupts 425
14.3 Interrupt Processing in the Protected Mode 426
14.4 Exceptions 430
14.5 Software Interrupts 432
14.6 File I/O 433
14.6.1 File Descriptor 433
14.6.2 File Pointer 433
14.6.3 File System Calls 433
14.7 Illustrative Examples 437
14.8 Hardware Interrupts 441
14.9 Summary 442
14.10 Exercises 443
14.11 Programming Exercises 443
15 Real-Mode Interrupts 446
15.1 Interrupt Processing in the Real Mode 447
15.2 Software Interrupts 448
15.3 Keyboard Services 449
15.3.1 Keyboard Description 449
15.3.2 DOS Keyboard Services 450
15.3.3 Extended Keyboard Keys 454
15.3.4 BIOS Keyboard Services 457
15.4 Text Output to Display Screen 463
15.5 Exceptions: An Example 464
15.6 Direct Control of I/O Devices 467
15.6.1 Accessing I/O Ports 467
15.7 Peripheral Support Chips 469
15.7.1 8259 Programmable Interrupt Controller 469
15.7.2 8255 Programmable Peripheral Interface Chip 471
15.8 I/O Data Transfer 472
15.8.1 Programmed I/O 473
15.8.2 Interrupt-driven I/O 475
15.9 Summary 480
15.10 Exercises 481
15.11 Programming Exercises 481
16 Recursion 486
16.1 Introduction 486
16.2 Recursion in Pentium Assembly Language 487
16.3 Recursion in MIPS Assembly Language 494
16.4 Recursion Versus Iteration 501
16.5 Summary 502
16.6 Exercises 502
16.7 Programming Exercises 502
17 High-Level Language Interface 506
17.1 Why Program in Mixed Mode? 507
17.2 Overview 507
17.3 Calling Assembly Procedures from C 509
17.3.1 Illustrative Examples 511
17.4 Calling C Functions from Assembly 516
17.5 Inline Assembly 518
17.5.1 The AT& T Syntax
17.5.2 Simple Inline Statements 520
17.5.3 Extended Inline Statements 521
17.5.4 Inline Examples 523
17.6 Summary 527
17.7 Exercises 527
17.8 Programming Exercises 527
18 Floating-Point Operations 530
18.1 Introduction 530
18.2 Floating-Point Unit Organization 531
18.2.1 Data Registers 531
18.2.2 Control and Status Registers 533
18.3 Floating-Point Instructions 535
18.3.1 Data Movement 536
18.3.2 Addition 537
18.3.3 Subtraction 537
18.3.4 Multiplication 538
18.3.5 Division 539
18.3.6 Comparison 540
18.3.7 Miscellaneous 541
18.4 Illustrative Examples 542
18.5 Summary 547
18.6 Exercises 547
18.7 Programming Exercises 548
Appendices 550
A Internal Data Representation 552
A.1 Positional Number Systems 552
A.1.1 Notation 554
A.2 Number Systems Conversion 555
A.2.1 Conversion to Decimal 555
A.2.2 Conversion from Decimal 557
A.2.3 Conversion Among Binary, Octal, and Hexadecimal 559
A.3 Unsigned Integer Representation 561
A.3.1 Arithmetic on Unsigned Integers 562
A.4 Signed Integer Representation 568
A.4.1 Signed Magnitude Representation 569
A.4.2 Excess-M Representation 570
A.4.3 1’ s Complement Representation 570
A.4.4 2’ s Complement Representation 573
A.5 Floating-Point Representation 574
A.5.1 Fractions 575
A.5.2 Representing Floating-Point Numbers 578
A.5.3 Floating-Point Representation 579
A.5.4 Floating-Point Addition 583
A.5.5 Floating-Point Multiplication 584
A.6 Character Representation 584
A.7 Summary 586
A.8 Exercises 587
A.9 Programming Exercises 589
B Assembling and Linking 590
B.1 Introduction 590
B.2 Structure of Assembly Language Programs 591
B.3 Input/Output Routines 592
B.4 Assembling and Linking 597
B.4.1 The Assembly Process 597
B.4.2 Linking Object Files 604
B.5 Summary 604
B.6 Web Resources 604
B.7 Exercises 604
B.8 Programming Exercises 605
C Debugging Assembly Language Programs 606
C.1 Strategies to Debug Assembly Language Programs 606
C.2 Preparing Your Program 609
C.3 GNU Debugger 609
C.3.1 Display Group 610
C.3.2 Execution Group 615
C.3.3 Miscellaneous Group 618
C.3.4 An Example 618
C.4 Data Display Debugger 620
C.5 Summary 625
C.6 Web Resources 626
C.7 Exercises 626
C.8 Programming Exercises 626
D SPIM Simulator and Debugger 628
D.1 Introduction 628
D.2 Simulator Settings 631
D.3 Running and Debugging a Program 633
D.3.1 Loading and Running 633
D.3.2 Debugging 633
D.4 Summary 636
D.5 Exercises 636
D.6 Programming Exercises 636
E IA-32 Instruction Set 638
E.1 Instruction Format 638
E.1.1 Instruction Pre.xes 638
E.1.2 General Instruction Format 640
E.2 Selected Instructions 641
F MIPS/SPIM Instruction Set 674
G ASCII Character Set 696
Index 700
More eBooks at www.ciando.com 0
Chapter 3
The Pentium Processor (p. 47)
Objectives
• To describe the basic organization of the Pentium processor
• To introduce the Pentium protected-mode memory architecture
• To discuss the real-mode memory organization
We discussed processor design space in the last chapter. Now we look at the Pentium processor details. We present details of its registers and memory architecture. Other Pentium details are discussed in later chapters.
We start our discussion with a brief history of the Intel architecture. This architecture encompasses the X86 family of processors. All these processors, including the Pentium, belong to the CISC category. Section 3.2 presents the internal register details of the Pentium processor. Even though the Pentium is a 32-bit processor, it maintains backward compatibility to the earlier 16-bit processors. The next two sections describe the protected- and real-mode memory architectures. Protected-mode architecture is the native mode for the Pentium processor. The real mode is provided to mimic the 16-bit 8086 memory architecture. In both modes, the Pentium supports segmented memory architecture. In the protected mode, it also supports paging to facilitate implementation of virtual memory. It is important for an assembly language programmer to understand the segmented memory organization supported by the Pentium. We conclude the chapter with a summary.
3.1 The Pentium Processor Family
Intel introduced microprocessors way back in 1969. Their first 4-bit microprocessor was the 4004. This was followed by the 8080 and 8085 microprocessors. The work on these early microprocessors led to the development of the Intel architecture (IA). The first processor in the IA family was the 8086 processor, introduced in 1979. It has a 20-bit address bus and a 16-bit data bus.
The 8088 is a less expensive version of the 8086 processor. The cost reduction is obtained by using an 8-bit data bus. Except for this difference, the 8088 is identical to the 8086 processor. Intel introduced segmentation with these processors.
These processors can address up to four segments of 64 KB each. This IA segmentation is referred to as the real-mode segmentation and is discussed later in this chapter. The 80186 is a faster version of the 8086. It also has a 20-bit address bus and 16-bit data bus, but has an improved instruction set. The 80186 was never widely used in computer systems. The real successor to the 8086 is the 80286, which was introduced in 1982. It has a 24-bit address bus, which implies 16 MB of memory address space. The data bus is still 16 bits wide, but the 80286 has some memory protection capabilities.
It introduced the protection mode into the IA architecture. Segmentation in this new mode is different from the real-mode segmentation. We present details on this new segmentation later. The 80286 is backward compatible in that it can run the 8086-based software.
Intel introduced its first 32-bit processor - the 80386 - in 1985. It has a 32-bit data bus and 32-bit address bus. The memory address space has grown substantially (from 16 MB address space to 4 GB). This processor introduced paging into the IA architecture. It also allowed de.nition of segments as large as 4 GB. This effectively allowed for a ".at" model (i.e., effectively turning off segmentation). Later sections present details on this. Like the 80286, it can run all the programs written for 8086 and 8088 processors.
Erscheint lt. Verlag | 28.9.2005 |
---|---|
Sprache | englisch |
Themenwelt | Informatik ► Programmiersprachen / -werkzeuge ► Assembler |
Informatik ► Theorie / Studium ► Compilerbau | |
ISBN-10 | 0-387-27155-4 / 0387271554 |
ISBN-13 | 978-0-387-27155-2 / 9780387271552 |
Haben Sie eine Frage zum Produkt? |

Größe: 3,7 MB
DRM: Digitales Wasserzeichen
Dieses eBook enthält ein digitales Wasserzeichen und ist damit für Sie personalisiert. Bei einer missbräuchlichen Weitergabe des eBooks an Dritte ist eine Rückverfolgung an die Quelle möglich.
Dateiformat: PDF (Portable Document Format)
Mit einem festen Seitenlayout eignet sich die PDF besonders für Fachbücher mit Spalten, Tabellen und Abbildungen. Eine PDF kann auf fast allen Geräten angezeigt werden, ist aber für kleine Displays (Smartphone, eReader) nur eingeschränkt geeignet.
Systemvoraussetzungen:
PC/Mac: Mit einem PC oder Mac können Sie dieses eBook lesen. Sie benötigen dafür einen PDF-Viewer - z.B. den Adobe Reader oder Adobe Digital Editions.
eReader: Dieses eBook kann mit (fast) allen eBook-Readern gelesen werden. Mit dem amazon-Kindle ist es aber nicht kompatibel.
Smartphone/Tablet: Egal ob Apple oder Android, dieses eBook können Sie lesen. Sie benötigen dafür einen PDF-Viewer - z.B. die kostenlose Adobe Digital Editions-App.
Zusätzliches Feature: Online Lesen
Dieses eBook können Sie zusätzlich zum Download auch online im Webbrowser lesen.
Buying eBooks from abroad
For tax law reasons we can sell eBooks just within Germany and Switzerland. Regrettably we cannot fulfill eBook-orders from other countries.